Category: Technology
Test-Driven Development (TDD): How to choose the Right Team To Drive TDD (Part 2)
Skills Needed
Technical and Analytical Skills of Business Analysts:- Ability to analyze a set of techniques and tasks to be used to work as a liaison among stakeholders and recommend solutions that enable the organization to achieve its goals.
- Collecting requirements and analyzing how they’ll work as a product. And what will be the future problems they may face. According to that unit tests are defined and the acceptance criteria are defined for further development.
- Writing a unit test before writing the code and able to write code sufficiently to make a failing test pass.
- Expertise in “test-driven bug fixing”, i.e. when a defect is found, writes a test exposing the defect before correction.
- Knows and can name several tactics to guide the writing of tests (for instance “when testing a recursive algorithm, first write a test for the recursion terminating case”).
- Able to factor out reusable elements from existing unit tests, through using situation-specific testing tools.
- Creating a “roadmap” of planned unit tests for macroscopic features (and revising it as necessary).
- Ability to “test drive” a variety of design paradigms: event-driven, functional, and object-oriented; Also the technical domains like persistent data access, user interfaces, and computation.
- Communicate properly with non-technical as well as technical people, especially explaining the technical challenges to the management team.
- Understand the client provided specs and visualize a real-time business situation. He/She must also be ready for a knowledge-sharing session with either his/her team or higher management every time the situation arises.
- Must also have a strong programming base to keep up with their team w.r.t. understanding what the client needs.
How To Pick the Right Team?
Evaluate your company’s methodology: If you’re a startup or an established firm looking for an overhaul in methodologies, then it’s important to determine what type of team you prefer that can successfully collaborate with your company. Check out websites and portfolios: A company’s website is the face you can judge it by. If the website works smoothly and the interface and user experience are user-centric, it means the company pays a great deal of attention to details. Assess the testing experience of the team: It is important to find out what types of testing a team can provide in general; what approach the team applies in each particular case, and understand what tests the team has performed on specific projects. Find out the industry focus of tested products: The quality assurance and testing team you hire needs to be experienced in testing apps or websites similar to yours. Compare the size/complexity of the company’s projects with yours: The size/complexity of the project also matters a lot. If a QA team has worked only on simple projects, it may not be ready to step up on a large and more complex project and test it comprehensively.Asking The Right Questions
In-depth questions will help you learn how they create and maintain quality assurance procedures, as well as filter out what meets your requirements. Here are some examples of questions you should ask: – What will TDD give us that we can’t get by building tests later? This question will help determine how well they understand and practice the benefits of TDD as mentioned above in the benefits section. – What quality assurance and testing process do you use and why? Gauge their understanding of QA processes and whether they have experience establishing these processes. This will also include the type of model they use to design the test plan. – What are the best practices, automation toolkits, and testing methodologies the team employs? This will enable you to understand the general testing approaches the team uses. Whether the team knows how to do automation testing, how they use automation testing, and with what tools they conduct it. – Do you use TDD for accessibility concerns as well? Since accessible features are getting a lot of attention, you can ask about their plans and involvement of accessibility concerns in your project. The concerns such as “does the correct ARIA hints are used for components?” and “is the correct semantic markup being used?” can be easily unit tested. -How will you evaluate that testing was successful? At this stage, specific expectations of testing may be revealed. Often, expectations don’t match that can be usually obtained through testing. In this case, it’s important to explain what exactly is impossible to do and why. -What will be the format for bug reports and testing results? This will help you agree on a specific format of bug reporting and testing results to ensure that you have all the information you need.Conclusion
Collaboratively discuss the complete project with the team and clear all the queries that you may have in your mind. Remember to be transparent, honest, and open about everything. Define your budget prior. Use the opportunity to build a personal connection with the offshore development agency. This rapport will decide to work with you in a better manner. Not only the Quality Assurance team, but you can also hire highly skilled developers, designers, testers, and digital product development experts. We follow agile methodologies like TDD to build robust custom applications for our clients. Contact us to know more about the development process and how we can help you to build and launch your application. About Galaxy Weblinks We specialize in delivering end-to-end software design & development services and have hands-on experience with automation testing in agile development environments. Our engineers, QA analysts, and developers help improve security, reliability, and features to make sure your business application and IT structure scale and remain secure.Test-Driven Development (TDD): How to choose the Right Team To Drive TDD (Part 1)
What Are The Expected Benefits of TDD?
Better designed, extensible, and cleaner code: It helps to understand how the code will interact with other modules and will be used. It results in more maintainable code and better design decisions. It helps in writing smaller code having single responsibility rather than monolithic procedures with multiple responsibilities. This makes the code simpler to understand. Reduced Scrap work and Time to Repair: TDD allows problems to be detected as early as possible, which has the effect of reducing the number of bugs. De facto, the technical debt is better controlled with a reusable, maintainable, and flexible code that allows the addition of new functionalities and reduces time to repair. Eliminates fear of change: Developers get quick alerts when a code change introduces a bug, and TDD’s tight feedback loop quickly notifies them when it’s fixed. Better code coverage: It provides better code coverage than writing tests after the fact. Because we create code to make a specific test pass, code coverage will be close to 100%. Faster developer feedback loop: Without TDD, developers are supposed to manually test each change to make sure that it works. With TDD, unit tests can run on-change automatically, allowing faster-debugging sessions and feedback during development.Common Pitfalls of TDD
Time-Intensive: While TDD generally results in higher-quality code, it is often a time-consuming process. But at the same time creating and maintaining a test suite, in addition to the software itself, is a significant investment. Many teams have seen significant reductions in defect rates, at the cost of a moderate increase in the initial development effort. Increased Overhead: The TDD process involves a great deal of overhead in the form of unit tests. Poor choices of testing, design, or architecture strategy at the early stage can be difficult to recover later in the project. Hence, it becomes difficult or impossible to make changes in the codebase without making dozens or hundreds of existing tests fail. Whereas, businesses that prefer to invest time in manual QA, or the ones that lack the technical resources to implement unit tests, are not the ideal candidates for TDD.Choosing projects for TDD
Existing Project (Legacy code) And New Projects A common TDD implementation problem rears its ugly head when an organization has inherited a system that wasn’t built with testability in mind. In such scenarios, questions arise like — Should the code be refactored? If so, how much refactoring is important to start practicing TDD in an achievable, and meaningful way? So, if the legacy code is already out there and working, the risk associated with the technical debt is low relative to the risk of new untested work. By applying TDD to the new code you’re writing, i.e., making changes in the existing code, you minimize the risk as well as don’t increase the technical debt.Microservices
Microservice-based architectures have far more complexities and dependencies than traditional application stacks. Creating tests for microservices-based applications and other complex architectures can be difficult due to the requirement for advanced stubbing and mocking. A microservice-based architecture, however, presents the opportunity to leverage TDD best practices in a very efficient way. The TDD pragmatist approach becomes very useful when you think about treating the microservice as the unit, rather than the compilation unit.Who should use it?
Most organizations simply do not have enough developers or time to cover all of their use-cases. This is especially true for enterprises that have a mix of skills and roles contributing to their projects. Leveraging TDD helps address the issue of limited technical resources by defining tests against the contract. With this approach, stakeholders, business analysts, testers, and other non-developer resources together can contribute to the TDD testing efforts since the Requirement Gathering phase.Conclusion:
TDD is a process that helps you start development projects with a healthy base of tests to ensure software quality throughout the development lifecycle. It helps you to produce maintainable, testable, and efficient code. But real-world conditions do not always make TDD adoption easy. It depends totally on your product requirement and can be made easier with the kind of team you have onboard. Contact us to know more about the development process and how we can help you to build and launch your application. In Part Two, we enumerated the skills and factors to consider while picking the best team. And we highlighted the right questions to ask the team to ensure how your requirements will be met w.r.t. the project. About Galaxy Weblinks We specialize in delivering end-to-end software design & development services and have hands-on experience with automation testing in agile development environments. Our engineers, QA analysts, and developers help improve security, reliability, and features to make sure your business application and IT structure scale and remain secure.The Quest Of The Most Extraordinary CMS - WordPress vs Drupal vs Craft
This article is an assessment and comparison of the popular content management systems WordPress, Drupal, and Craft. We’ll dig into each CMS platform’s features, ease of use, the types of businesses that are best suited to the platform, and more. We’ll also walk you through the use case scenario of each CMS and will help you understand which one could be right for your business and goals.
Before we explore the nuances of each CMS, let us quickly introduce the three stalwarts in the CMS arena.
WordPress
WordPress is an extremely versatile CMS that facilitates users (even non-developers) to create feature-rich websites and ecommerce portals. It is completely customizable, mobile-optimized, and SEO friendly. It’s also open-source, making it completely free, redistributable, and offers unlimited validity.
The most popular CMS platform powers more than 40% of the websites available on the internet. It also offers thousands of free and paid themes and plugins that the developers can choose from.
Drupal
Drupal’s open-source model encourages continuous improvement and innovation through the support and passion of the Drupal community. With a pool of diverse experts regularly working to make Drupal better, the possibility to create exceptional digital experiences expands far beyond what a single team of proprietary software developers could dream up.
Recent versions of Drupal have also prioritized user experience (UX) to empower everyone from the site editors to content creators and to build powerful customer experiences.
Craft
Craft CMS is a content management system similar to WordPress. The interface is a simple, more stripped back version of WordPress, built and tailored to the needs and requirements of the business. This simple view makes updating and adding content a much more seamless experience.
For some, this adds an increased level of comfort when migrating over to Craft due to the out-of-box understanding developers, designers, and writers are likely to have.
Comparison — WordPress, Drupal, & Craft CMS
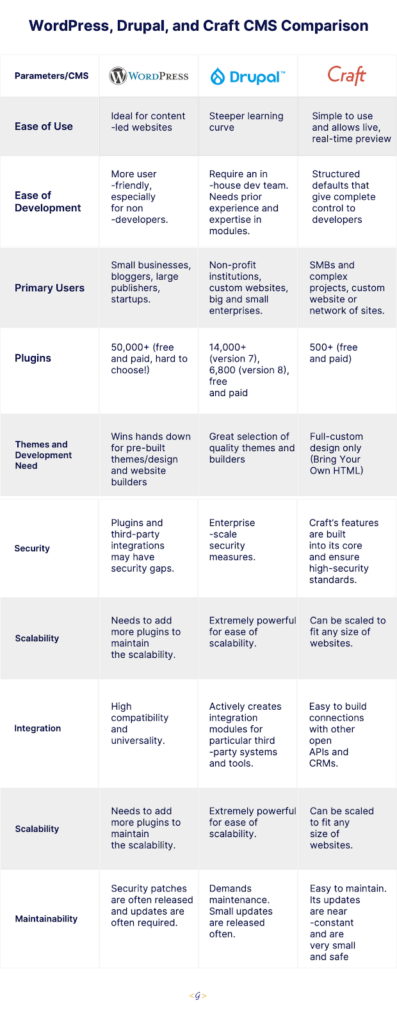
Below are the brief use cases for each CMS, showcasing when and how the three of them are being used to help you decide which one suits your business needs, size, and scalability.
WordPress
One of the common misconceptions about WordPress is that it’s mainly for creating blogging sites. WordPress was developed as a blogging platform, but that is ancient history now. The platform has evolved radically with the various new releases over the years.
The good news with WordPress is that it is extremely flexible. It can be adapted for ecommerce websites, corporate websites, communities, and forums. WordPress is an ideal fit for startups as well, as it allows them to start small and then scale up to run even enterprise level organizations that span many countries and continents.
All you need are suitable plugins and themes. So, for instance, if you wanted to make an online portfolio for your web design business or even a blogging site, you could go with the provided themes. You can alter its source code to customize your website’s look or functionality!
You have a choice of adding extra oomph to your website by opting for customizations.
For example,
You may have a website that includes a design that should be converted to HTML, CSS, and JavaScript — being three different “technologies”, and be mobile friendly as well. That design is then integrated into a WordPress theme. Whereas all dynamic components such as menus, sidebars, footer widgets, and general content areas should be connected and coordinated with the backend.
Several custom features are then built as custom plugins by a back-end developer, who should be aware of the front-end specifics and business needs through a manager. That final product is packaged and then hosted on a specific server environment.
It will require an extra pair of hands further when it comes to security (having more people able to tackle an assignment and a project manager in charge), having potentially higher code quality when a bunch of people carry out code reviews. This process includes automated testing and ongoing scalability given the diverse expertise of our WordPress developers.
In addition to that, WordPress might be free but it is common for businesses to pay the experts to design a logo for you or adjust some of the CSS code on your site. Whereas, other WordPress users are keen on keeping graphic designers or maintenance experts on call.
So, it all depends on your experience and the scale of your website.
Drupal
Drupal is the best choice if you are building a website that needs complex data organization, that needs to be customizable.
For instance, when you’re building an advanced website, you can choose from among many technologies. Usually, the first choice is the programming language (ASP.NET, Java, Python, PHP, etc.) and then we decide whether we should code everything from scratch or use a framework to do all the heavy lifting.
There is also a third choice, a more comprehensive solution, CMF (content management framework). Drupal 9 is one of these frameworks/systems.
And by large and advanced, we mean one that is changed frequently. Content changes are carried out by at least one editor, supported by at least one developer (along with a QA tester and a sysadmin), who’s responsible for fixing the bugs, continuous development of the website, adding new functionalities, and so on.
Some examples of large and advanced websites are:
- A corporate website of a medium or large production or service company.
- An informational website, for example, run by a paper or magazine.
- An organization’s employee portal for managing processes in the company, such as a CRM system.
- A system feeding content to other systems — an API with content for other websites and mobile applications.
Besides, Drupal has almost everything you need out-of-the-box. But more often than that, you may need a functionality that is not a part of the Drupal core yet. And to fulfill that need, you need modules. Modules are bundles of code that can extend or add the functionality of Drupal websites. For example, Drupal Commerce provides everything required to sell products, services, or files online.
Craft
With Netflix, Moz, Duck Brand Duck Tape, Salesforce, PBS, and countless other brands running Craft websites, it’s a mature and competent content management system. At the same time, one of the most important things about Craft is that it is among the newest CMS solutions.
Craft CMS has ditched the traditional CMS systems that come with pre-built themes, CSS frameworks, and ready-to-go websites. It provides a bespoke solution to developers. Craft CMS neither offers posts or pages nor does it offer any bootstrap features and themes to spin up the frontend.
Everything is coded and designed from scratch which allows creating a unique experience specific to your project. And a fluent, sensible content management experience is the result.
Craft specifically targets developers responsible for building complex websites for companies with large budgets.
The primary reason for what makes Craft successful is that CraftCMS gives web professionals and developers control over websites they build.
There are many key tools in CraftCMS that are managed through the control panel of the CMS that illustrates how Craft supports and favors developers of your business:
- Hundreds of Craft mediated plug-ins
- Live preview during the website build
- Regular releases and bug fixes
- Complete control over the content and categories
- An ability to manage multiple sites with one Craft installation
- Custom templates
- Allowing developers to write and use their own HTML
Why does it matter to your business?
When we boil this down to value for your business, all three CMS ensure a robust and feature-rich website with fewer maintenance costs, faster publishing, and quicker onboarding of new people.
The flexibility and features they offer are out of the box, along with their content-first approach, and make them our go-to for nearly every custom web project.
It’s a crucial part of our web design and development capabilities. Intrigued? Get in touch with us and we’ll be happy to talk about how they can make your site great.
Best Frontend Development Frameworks for 2021
React
React makes it easy for developers to create interactive user interfaces. The framework is designed with backward compatibility and component-centric applications in mind, so you can be assured about the longevity of your application. One of its major advantages is the ability to use it for native development. Reusable components, better performance, support from Facebook, a large developer community, and SEO friendliness, are some of its added advantages. When To Use React:- Developing small enterprise-level apps
- Creating SPA or cross-platform applications
Angular
Angular is generally used to build front-end applications in both large enterprises and small-sized companies. However, its popularity has taken a dip in the past few years. Angular increases the performance of browser-based applications by dynamically updating the contents in no time since it uses two-way data binding. When To Choose Angular- Large scale applications
- If you need a scalable architecture or prefer TypeScript
- Building real-time applications
Vue
One of the most popular front-end frameworks nowadays! Vue is a straightforward and simple framework. It’s good at removing the complexities that Angular developers face. It’s smaller in size and offers two major advantages – a visual DOM and a component-based approach in building user interfaces. It’s also a 2-way binding ecosystem that can handle both dynamic and simple processes with ease. Why Choose Vue.js:- Helps design everything from scratch and is successful in developing giant projects as well
- Assists in multiple tasks
- Helps in building web applications, mobile apps, and progressive web applications
Ember.js
Ember.js is an open-source JavaScript framework. It is used to create scalable, enterprise-grade, single page web applications. It provides custom properties, useful binding, and ready configuration to render the page as needed. Even though Ember is one of the older front-end frameworks compared to Svelte, Vue, and React, it still has a big user base and packs a punch. Reason to use Emberjs:- Well-organized
- Fastest framework
- Two-way data binding
- Companies like Microsoft, LinkedIn, Netflix, Twitch, etc. are among its clients
Svelte.js
Svelte.js is an open-source, component-based frontend JavaScript framework written in Typescript. It is touted as a game-changing and revolutionary idea. Svelte, unlike React or Vue, has no virtual DOM and does not require high browser processing. Instead, you build components boilerplate-free in simple JavaScript, CSS, and HTML code. Reasons to choose Svelte.js:- Lightning-fast apps with excellent performance
- Zero client-side dependencies, and no need for complex state management libraries
- Used by Godaddy, Razorpay, 1password, New York Times
Backbone.js
Backbone.js is an easy-adoption framework that lets you develop SPAs swiftly. It’s based on the MVC architecture. The platform also allows you to develop projects that require different types of users, where the arrays can be used to distinguish the models. So, regardless of whether you intend to use Backbone.js for backend or frontend, this is an ideal choice as its REST API compatibility offers seamless synchronization between the two. Reasons to choose Backbone.js are as follows:- Backbone.js is used for dynamic applications like Trello.
- Helps in building client-side models, updating faster, and reuse the code.
- Easy to learn
- Lightweight framework
Preact.js
Preact.js follows a component-based approach with Virtual Dom, the same as React – while being completely compatible with React. It can even use React packages without compromising on leaner size, performance, and speed. In cases where the full potential of React isn’t necessary, most developers even use React during development and switch to Preact for production. Reasons to choose Preact.js:- Lightweight, swift, and high-performance library
- Simply 3kb in size (minified and zipped) and yet gives you all the necessary functionality of React
- Many major companies like Lyft, Uber, and Tencent are using Preact
A brief overview of the React 17
Changes in event delegation
The React 17 update eases the nesting of applications that are built using different React versions. A lot of conflicts were seen when many versions of React are being used together. With this update, event handlers will not be attached at document levels. In its place, it will go to the root DOM container where your React tree is rendered. Also, with React 17 going in for gradual changes, developers will have more freedom when it comes to migration of apps in one go or smaller chunks.Native component stacks
Finding errors with javascript function; its name and location stack trace can lead to a needle in a haystack hunt while sifting through hundreds of coding lines (if not a thousand). To pinpoint errors in React 17, there is an unorthodox approach taken by the React team. Here is what they are thinking, in their own words:Currently, the browsers don’t provide a way to get a function’s stack frame (source file and location). So when React catches an error, it will now reconstruct its component stack by throwing (and catching) a temporary error from inside each of the components above, when it is possible.This improvement will come in handy during the production environment.
No More Private Exports
The new update of React has withdrawn private exports. This was a much-awaited update for React Native for Web. The dependency of React Native for Web was on certain internals of the event system. This dependency was fragile and now will be addressed with this update.No Event Pooling
Event pooling optimizations are no longer a part of React 17. It failed abysmally to enhance the performance in older browsers. Its initial purpose was to reuse the event objects between events along with setting all fields to ‘null’ between processes. However, it ended up causing more confusion for developers. Following this change, the need to use e.persist() is also eliminated.Properties of React Components
React 17 is now compliant with ES6. There are talks of using key or codes and in case you are working with it, you are required to code property to keyboard event objects. On similar lines, capture phase events use real browser capture, there are no bubbles in onScroll events, etc. In addition to the above-mentioned updates, two new functions are being introduced in this update, namely ‘getDerivedStateFromProps’ and ‘getSnapshotBeforeUpdate’. All this said, React 17 is surely working towards making React more prominent within the developer community and making it easier for all to understand, deploy, and migrate to React in the future. Feel free to get in touch with us here if you need help with your React development. About us We, at Galaxy Weblinks, are all for new ideas and experiments. We believe that being up-to-date in this fast-paced world gives us adequate time to explore and implement new changes. Our developers are constantly striving for improvement and a better user experience. Contact us for a free consultation!Migration Plan To The All New Vue JS 3
Vue 3 is here and everyone is looking forward to catching up with the upgrade ASAP.
Vue JS is the best client-side framework out there! It has improved its bundle size and performance under the hood, making this new version appealing to the developers.
What’s the catch? Some breaking changes, deprecations, and new syntax might make your migration plan slightly harder than expected. Let’s dive in and see what you should expect.
In this article, we’ll look at how you can upgrade your Vue.js 2 application into Vue 3, and what are the breaking changes you should expect in Vue 3.
How to upgrade from Vue 2 to Vue 3
Let’s assume a scenario where you have a usual Vue.js project. You would also have a main.js file where you import the Vue router and Vuex configuration and set it to the Vue instance.
Using the CLI
Now to upgrade a Vue.js 2 project to Vue.js 3 project, you can simply add an official CLI for Vue projects, the Vue-next plugin. Besides deployment and development tooling, it simplifies the upgrade to a one-line command:
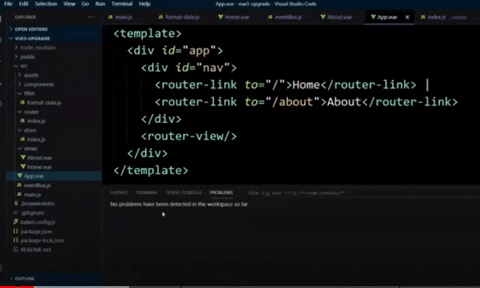
This plugin upgrades and installs the new dependencies for Vue Router, Vue, and Vuex. It also modifies the code to be compatible with Vue 3 version.
So it becomes important to create a backup of your project at the very start.
Installing Dependencies
Installing the plugin upgrades the packages @vue/test-utils, eslint-plugin-vue, vue-router, vuex, and vue to the new version. Also, a new package appears among the development dependencies, named @vue/compiler-sfc.
It helps in compiling the new Vue single file components into runnable Javascript code. Now, you can use your source control panel to see and compare all the changes.
Code Comparisons
The first thing you will notice is that the way of instantiating and configuring your application has changed. It looks and works much better than before.
Same for the Vue router. If you take a look at the configuration file, a new VueRouter becomes a call to createRouter, and the global plugin setup must be left. Instead, you’ll be using some functions from this library.
The same goes for the Vuex Store. You can use the new ‘createStore’ function to create a new instance and set the store configuration.
Breaking Changes You Would See in Vue 3
The above modifications are done automatically by the Vue CLI.
All you have to do now is to start experimenting with all the below new features that Vue 3 offers for which you will need experienced developers. Let’s understand this better.
Vue Filters and Eventbus are deprecated in the new version. EventBus was there in the Vue development whenever you’re facing a situation where you need to make a shortcut to emit events from a child to parent, or vice versa. Whereas, filters were there to help you ‘format’ your value, for example: to short URL, add currency, uppercase, etc.
You will need experts in complex cases to make the required changes in your application code and refactor both of them.
You should expect a more maintainable, smaller, faster version with the new features. Most of them are additions and improvements over the existing API.
Composition API
Composition API is Vue 3’s biggest feature so far, which provides an alternate syntax for using TypeScript, reusing code across components, and writing larger components.
This is an alternative syntax and not the new way we code components in Vue 3. You might choose to use it based on the above three reasons.
Another good thing is that the existing Options API is not deprecated. Everything is for handling advanced use cases and mainly replace mixins usage that admittedly has caused a lot of problems in large scale applications.
Mounting
As we mentioned before, the first thing that you will notice is the difference in initializing your app. In Vue 2 you use Vue constructor with the $mount method and a render function like this:
In Vue 3, you will find a more elegant syntax
Fragments
In Vue 2, multi-root components were not supported. The solution was to wrap your code in a wrapper element.
In Vue 3, components can have multiple root nodes now. This removes the use of wrapper elements and enables writing a cleaner markup.
Teleport
Having part of your component mounted in a different position in DOM than the Vue component hierarchy has been seen as a difficult situation to solve.
The teleport feature helps in creating a component that includes a full-screen modal. In most cases, you’d want the modal’s logic to live within the component, but either it starts requiring a change in component composition or the positioning of the modal quickly becomes hard to solve through CSS.
You can still interact and pass props to it like being inside the component!
Conclusion
If you want your Vue.js application to become faster, smaller, more maintainable, you should upgrade to Vue 3 with an easier approach to target native.
If you want to wait for Vue.js Migration Build release for the whole upgrade process, you can still stick to using Vue.js 2, as it’s stable and it works with any third-party library or tool.
If planning for migration, we recommend that you hire experts who can assist you with a proper migration plan and ensuing and development process, especially as the supporting libraries are still getting updated.
Contact us for your new custom or single-page application Vue.JS project.
About Galaxy Weblinks
We specialize in delivering end-to-end Vue.js software development services, apart from other popular JS frameworks. Our team of skilled and dedicated Vue.JS developers provides initial consultation, migration plan, and the final Vue.JS web app development & support. We deliver powerful front-ends, real-time applications, and single-page apps (SPAs) with on-time delivery with an agile & devOps approach.
3 Cloud Problems That Needs Your Attention
Cost management
Most businesses would agree that cloud providers keep changing their billing practices, adding unwarranted complexity to what is supposed to be a fairly simple thing. When you look at all the possible configurations it’s easy to get lost in the services enlisted in the invoice by your provider. It’s not that just providers are at fault here! Businesses often make several mistakes that can increase their expenses. Sometimes, IT professionals like developers turn on a cloud instance implied to be utilized temporarily and then forget about it later. If you cannot make sense of your bill, what you save on the infrastructure will be lost on bandwidth and other hidden things.Compliance
Enterprises use the cloud to store all sorts of information, personal and otherwise. With all that information and migration of this information, GDPR compliance poses a challenge. While handling complex cloud environments, there is little time for organizations to worry about the implementation of GDPR. Any breach of the compliance and the business goes under. Add to this mix the fines which can range from 2-4% of the company’s annual revenue, if found violating the law. Many organizations turn to employ a data protection professional who can anticipate data security and privacy according to the needs of the law. These professionals are aware of the compliance needs of the organizations they are employed in, concentrating on the duties for compliance will help organizations fulfill every legal responsibility.Cloud Security
According to a Unisys-sponsored survey, 64% of U.S. Federal Government IT leaders view identity management solutions as critical to cybersecurity. When we talk about security, we’re just scraping the surface of the cloud concerning what we know about the cloud and how to secure it. Furthermore, the cloud providers do not give us any choices besides using their native security solution the platform comes equipped with. A recipe for a complex system we must add. IAM or Identity Access Management means seamlessly controlling access and rights for every user on the network. Almost every enterprise has IAM best practices in place. However, they are only effective if strictly followed across the organization. Unchecked or mismanaged exceptions and exemptions to IAM policies are some of the leading causes of compromised data. Multifactor authentication is our best bet at securing our clouds and will eventually become ubiquitous.To Conclude
When compared with the benefits, the cloud limitations seem to get dwarfed. However, there is still a lot of work that needs to be done by both – the services providers as well as the enterprises. Organizations can steer clear of these challenges if they have verified cloud experts by their side to guide them through. Need help with your cloud implementation?? Let us help you. About Galaxy Weblinks Galaxy has a proactive cloud team that works round the clock to deploy and ensure the safety of the systems across various clouds like AWS, Google Cloud, and Microsoft Azure.Flutter 1.22 Update| All You Need To Know
Flutter iOS 14 Updates
iOS app development is now set to be a better process than before with Flutter 1.22 update. There is now support for XCode 12 and as Xcode requires iOS 9.0 or higher versions, the default template is now 9.0 (previously it was 8.0). Developers are getting preview support for App Clips too. Crashes reported specific to iOS and font rendering issues were also fixed in this update. And not to miss, when a developer is using cupertino_icons 1.0 with Flutter 1.22, they are getting access to almost 900 new icons via the CupertinoIcons API.Flutter Android 11 Update
There are two updates for Android developers. Flutter now supports exposing the safe insets of various Android displays i.e. notches, cutouts, and the display of waterfall edges. This will facilitate the placement of elements via MediaQuery and SafeArea APIs. And the second one is that animations are now in synchronization with the Android keyboard.Google Maps And WebViews
Both these plugins are now ‘production ready’. One can write the code, build the APK, and release it, as per convenience. There is a note here by the Flutter team. They say that Android developers will have to manually enable webview_flutter until it becomes a default setting.Extending The Buttons Family
Keeping up with the Material guidelines, there is now an additional ‘universe’ of buttons. Now developers won’t have to make widgets from scratch because of the lack of a few buttons. One can mix and match the older and newer buttons as per their requirements.Analysis Tool For App size
Your developers won’t be quizzed for app sizes ever again. They can get their hands on the breakdown of native codes, assets, and even package level details of the compiled dart code. This size and composition summary is given by the analysis size tool. Such insights will help your team for keeping the app size within the set limits.Smooth Scrolling
This feature is still in preview but it is an interesting one. It is present to bridge the gap between input and the display frequencies when they are not the same. Enters, resamplingEnabled flag. Flutter claims that you can see almost 97% improvement by enabling this flag and removing said discrepancies. Once there is a certain consensus about this feature, you can expect it to be the default setting. Apart from the above-mentioned features, there are some notable ones listed below:- Support for new internationalization and localization
- Improved navigator 2.0
- Improvement in the output linking of VSCode
- State Restoration option in Android (In Preview)
- Developers can host the inspector tab from Dart DevTools directly inside IntelliJ.
About us
We, at Galaxy Weblinks, are all for new ideas and experiments. We believe that being up-to-date in this fast-paced world gives us adequate time to explore and implement new changes. Our mobile development team is proficient in building apps that are well aligned to our client’s and platform requirements. Contact us for a free consultation!How to get Bidirectional Scrolling right in your responsive design
Incessant scrolling while using an application is perceived as a flaw on both mobile and desktop. To address this concern, a shift to horizontal scrolling of elements is in trend now.
We are seeing an increase in designs using non-breaking lists that are scrolled horizontally for more content especially on mobile, where horizontal space is limited.
On the other hand, vertical scrolling works as a mainstay of virtually every digital interaction you’ll come across.
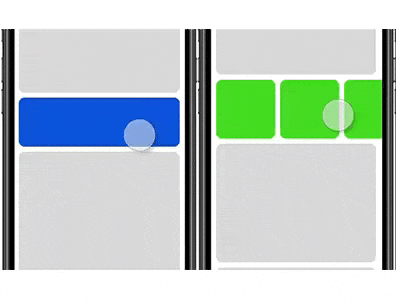
A few years ago, the users used to remain reluctant to move sideways through content. However, the users have evolved since then and are more receptive to the idea of scrolling in both directions. It is more preferable to scroll through vis-a-vis clicking multiple pages or screens. Scrolling is completely natural and more efficient.
Users have learned to scroll. Never-ending newsfeeds and content have taught this interaction to users over and over again. In a mobile-first world, scrolling has become the number one interaction with digital content.
Users are impatient. They don’t wait to get a page fully loaded to scroll and discover the content later.
Although, the whole shift has led to the challenge of designing a smooth bidirectional scrolling experience without compromising the responsive design.
- Creating desktop and mobile experience a mess while trying to ensure the same user experience
- Hiding content behind menus
- Having to scroll down and across in a zig-zag fashion
- Taking more clicks (or flicks) to horizontally scroll the content into view on desktop
Here are two best practices for bidirectional scrolling to figure out when horizontal scrolling is great for UX:
Horizontal scroll works best when you want to display a subset of a category
Websites like Netflix have been using the responsive principle to showcase huge libraries in a way that lets you get to what you’re interested in extremely quickly.
Even e-commerce shoppers are scrolling more frequently than ever and are doing a great job when it comes to responsive design.
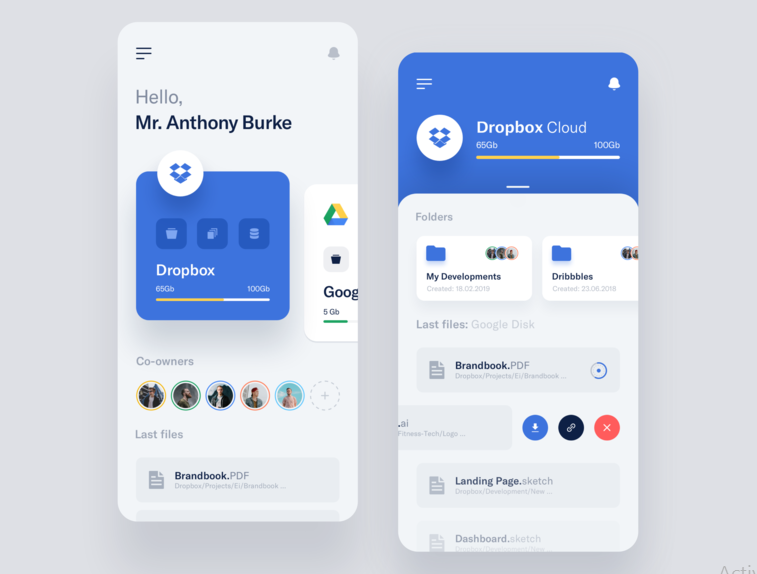
Image source: Vlad Ermakov for widelab
You’ll see a section, and there’s a plethora of content towards the right if you keep scrolling. When you scroll down, it’s just one line for each topic or section, making it easy to get to what you want quickly, then scroll to the right to dive deeper into that section.
The main principles that these sites have in common and have seen success with are:
- Displaying a large catalog of products or items so that different product categories can be easily shown.
- Displaying discrete sections or slides of information on applications like recommended or similar products on e-commerce websites.
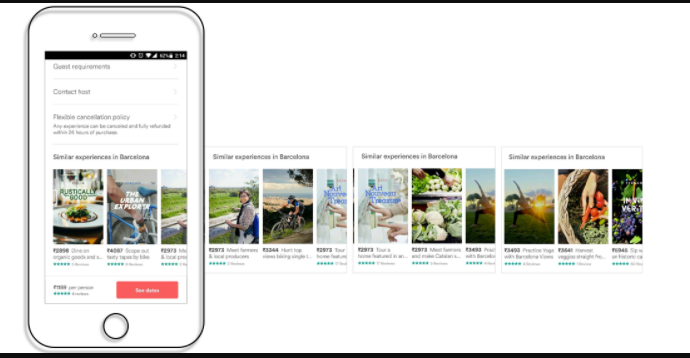
Make your navigation more obvious and attractive
This seems to be the biggest problem faced by visitors when it comes to horizontal scrolling based websites. Even those that look incredible can end up confusing and turning off their users. That will result in leaving your website and finding one that is easier to get around on.
So navigation should be clear and easy so that your users don’t have to sit on your page for too long trying to determine how to get somewhere.
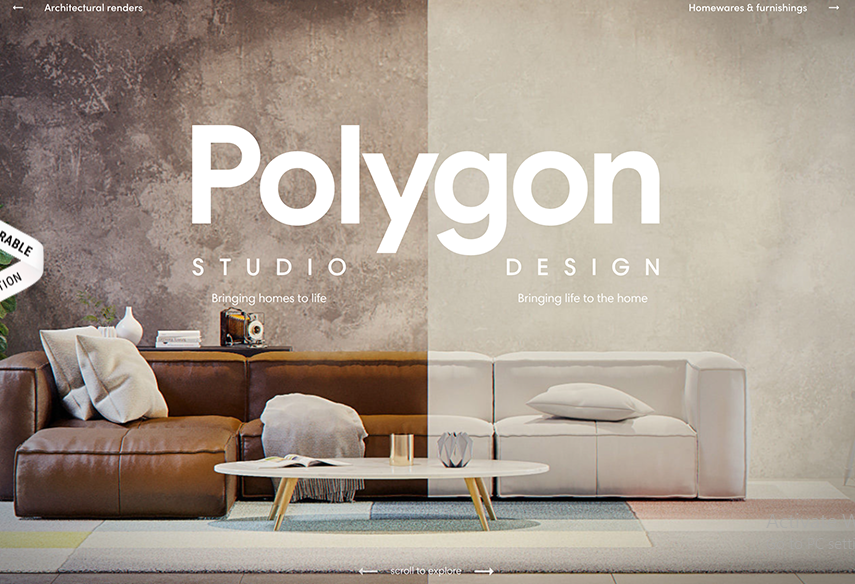
Make sure there is a visible label, and easy to use menu options of some kind that will get them to the information they want, especially about the bidirectional scrolling at the home page.
“Users are encouraged to scroll when it’s easy for them to see what is visible on the page without even requiring any action.”
If you fail to provide the right e-commerce site elements and above the fold and create an enjoyable digital shopping experience, you’re going to have difficulty getting shoppers to scroll.
The honest truth is that you need just the right mix of vertical and horizontal scrolling.
Conclusion
There is a lot of debate over the internet about the usage of the bidirectional scroll and its impact on UX. But it helps a lot in compartmentalizing the content and saves a lot of vertical space on an app if used wisely.
If your app and website are struggling with responsive design issues, feel free to contact our experts.
About Galaxy Weblinks
We specialize in delivering end-to-end software design & development services. Our design process stems from an agile and a responsive digital development that incorporates effective collaboration, streamlined projects which strives for better results.